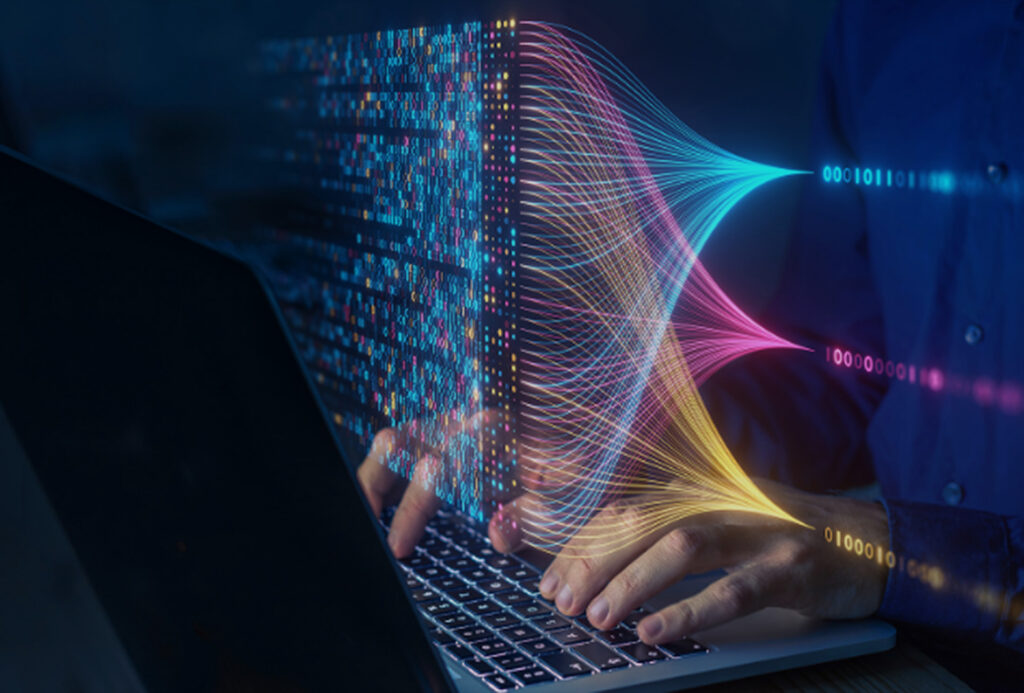
SAP ABAP Fundamentals Course
Course Overview
This SAP ABAP (Advanced Business Application Programming) Fundamentals course is designed for individuals who are new to ABAP programming and would like to develop a strong foundation in SAP development. The course covers the essential concepts, tools, and techniques required for writing ABAP programs in SAP, including understanding data structures, creating reports, managing database tables, and integrating with other SAP modules.
Course Objectives
- Understand the basics of ABAP programming language.
- Learn to create simple reports and manage database tables.
- Understand data types, control structures, and modularization techniques.
- Gain the skills necessary to debug ABAP programs and troubleshoot errors.
- Integrate ABAP programs with other SAP modules.
Module 1: Introduction to SAP and ABAP
- What is SAP?
- Overview of SAP ERP and its modules
- The role of ABAP in SAP
- Key features and capabilities of ABAP
- ABAP Development Tools (ADT)
- Introduction to Eclipse-based ABAP Development Tools
- Navigating the SAP GUI and ABAP Workbench
- ABAP System Architecture
- SAP NetWeaver and its components
- The ABAP runtime environment
Module 2: Getting Started with ABAP
- ABAP Syntax and Structure
- Basic syntax, keywords, and statements
- Understanding ABAP programs and their structure
- The significance of the INCLUDE statement
- Writing Your First ABAP Program
- Creating a simple “Hello World” program
- Understanding the WRITE statement for output
Module 3: Data Types and Variables
- Data Types in ABAP
- Elementary data types: CHAR, NUM, DATE, TIME, etc.
- Structured data types: TABLES, STRUCTURES
- Complex data types: Internal Tables, Work Areas
- Declaring Variables
- Using DATA statement
- Constants and literals
- Working with fields and data objects
- Internal Tables and Work Areas
- Introduction to internal tables
- Declaring and filling internal tables
- Using loops to process internal tables
Module 4: Control Structures
- Conditional Statements
- IF, ELSEIF, ELSE
- CASE statement
- Loops and Iteration
- DO, WHILE loops
- LOOP AT statements for internal tables
- Error Handling
- Using TRY…CATCH to handle exceptions
- SYSTEM fields and ABAP error messages
Module 5: Modularization Techniques
- Subroutines (FORM and ENDFORM)
- Creating reusable subroutines
- Parameter passing between subroutines
- Function Modules
- Introduction to function modules in ABAP
- How to create and call function modules
- Using standard SAP function modules
- Methods and Classes (Object-Oriented ABAP)
- Introduction to Object-Oriented Programming (OOP) concepts in ABAP
- Creating simple classes and methods
Module 6: Working with Database Tables
- Database Access in ABAP
- Introduction to Open SQL and Native SQL
- SELECT, INSERT, UPDATE, DELETE statements in Open SQL
- Using internal tables for database operations
- Database Table Structures
- Understanding database tables and their structures
- Primary and foreign keys, indexes
- Selecting data from database tables
- ALV (ABAP List Viewer) Reporting
- Introduction to ALV for displaying data
- ALV Grid and ALV Tree Control
- Customizing ALV output
Module 7: Developing Simple Reports
- Classic Reports (List Reports)
- Creating simple report programs
- Using SELECT statement for data retrieval
- Formatting output with WRITE and LOOP
- Interactive Reports
- Handling user interaction with reports
- Using event blocks (AT LINE-SELECTION, AT USER-COMMAND)
- Navigating between report lists
Module 8: Debugging and Troubleshooting ABAP Programs
- ABAP Debugger
- Introduction to ABAP Debugger
- Setting breakpoints and watchpoints
- Analyzing variables and program flow during runtime
- Error Handling Techniques
- Using error messages and exceptions
- Log files and dumps
- Working with ST22 and SM21 transaction codes
Module 9: Enhancements and Exits
- User Exits and BADI (Business Add-Ins)
- What are user exits and BADIs?
- Enhancing standard SAP functionality without modifying the core system
- Implementing custom business logic through exits and BADIs
- BAPI (Business Application Programming Interface)
- Introduction to BAPIs
- How BAPIs are used for data exchange between systems
- Calling BAPIs from ABAP programs
Module 10: Best Practices and Performance Tuning
- Writing Efficient ABAP Code
- Understanding performance pitfalls and optimizations
- Efficient handling of internal tables
- Avoiding nested SELECTs and optimizing database access
- Code Optimization Tips
- Minimizing the number of database calls
- Using buffered tables and indexed searches
- Best practices for memory management and data buffering
Module 11: ABAP Integration with Other SAP Modules
- RFC (Remote Function Call) and ALE (Application Link Enabling)
- Introduction to RFC for cross-system communication
- Using ALE for data distribution between systems
- IDocs (Intermediate Documents)
- What are IDocs and how they are used for data transfer
- Working with IDocs in ABAP programs
Module 12: Course Wrap-up and Project
- Real-world ABAP Project
- Applying the concepts learned to a real-world scenario
- Project completion with debugging, optimization, and reporting
- Review and Q&A
- Recap of key topics covered
- Open forum for participant questions and feedback
- Certification
- Final assessment to gauge understanding of ABAP fundamentals
- Issuance of course completion certificate
Course Delivery Method
- Format: Blended (Lectures, Hands-on labs, and Projects)
- Tools: SAP GUI, Eclipse, ABAP Workbench
- Duration: 4-6 weeks (depending on pace)
Target Audience
- Beginners in ABAP programming
- SAP technical consultants and developers
- Individuals seeking to enhance their skills in ABAP development
Pre-requisites:
- Basic understanding of programming concepts (preferably object-oriented programming)
- Familiarity with SAP software (SAP GUI)
Conclusion
By the end of this course, participants will have a solid foundation in ABAP programming and be well-equipped to develop, debug, and optimize SAP applications. Whether you’re pursuing a career as an SAP developer or simply looking to enhance your technical skill set, this course will provide you with the necessary knowledge to succeed in ABAP programming.